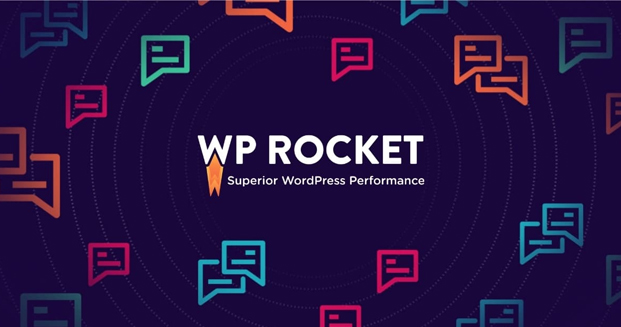
With Google’s new PageSpeed update called “Core Web Vitals”, having a fast-loading website, or at least, a website that appears to be fast-loading, is very important. Lazy loading is great for images that are off of the screen, but blog posts typically have an image at the very top of the post, so lazy loading these images can cause problems.
Enter WP Rocket. WP Rocket lazy loads images, and has several ways to disable lazy loading on specific images. The problem is, all of your images likely share a similar CSS class, so the methods in the current version of WP Rocket will not let you disable a specific image in your post content programatically.
Since your first image in your post doesn’t load until the entire page is loaded, this hurts your “First Contentful Paint” score in Core Web Vitals. They want to see that image show up as quickly as possible to give it the appearance that it is loaded more quickly for user experience – even if your site is technically loading faster, it appears to be loading slower if your above-the-fold images are lazy-loaded.
What we need to do to improve your score is only disable lazy loading on the first blog image.
If this sounds like something you’re looking for, my code might be of some use to you. I’ve searched for a solution with no success, but I found a handful of other people looking to do the exact same thing.
So, I wrote some code to make this work (add to your functions.php file in your theme):
/**
* Disable lazyloading from first blog image
*/
function add_responsive_class($content){
if ( is_single() ) {
$content = mb_convert_encoding($content, 'HTML-ENTITIES', "UTF-8");
$document = new DOMDocument();
libxml_use_internal_errors(true);
$document->loadHTML(utf8_decode($content));
$imgs = $document->getElementsByTagName('img');
$img = $imgs[0];
if ($imgs[0] == 1) { // Check if the post has images first
$img->setAttribute('class','alignnone size-full remove-lazy');
$html = $document->saveHTML();
return $html;
}
else {
return $content;
}
}
else {
return $content;
}
}
add_filter ('the_content', 'add_responsive_class');
function rocket_lazyload_exclude_class( $attributes ) {
$attributes[] = 'class="alignnone size-full remove-lazy"';
return $attributes;
}
add_filter( 'rocket_lazyload_excluded_attributes', 'rocket_lazyload_exclude_class' );
Here’s what this does:
1. Only apply this to blog posts. If you have custom post types, you may want to replace this “is_single()” line of code to something that only focuses on posts in specific categories, with something like this:
if(in_category(array('1', '2', '3', '4', '5')) ) {
2. The content is loaded, decoded, and the images are found.
3. If the post has images, it will take the first image (or image #0) and add the class “remove-lazy” to it, so we can disable lazy loading with this unique class name later.
4. If the post does not have any images, it just returns the content as it normally would.
5. The next filter is taken from the above link on WP Rocket’s website. It excludes lazy loading on any image with the class “remove-lazy”.
If you don’t use full-size images in your WordPress posts, or you align your images differently, you might have to tweak some of these lines that say “alignnone” and “size-full” to match the settings that you use for your first image in your blog post. In my case, I never add custom alignment and I always use full-size images.
You can see this in action on any post on my company blog, here.
If this helped you, if I missed anything, or if you have anything you’d like to add, please let me know in the comments below!
Hey there, I really found this post useful for my blog. I used to use W3 total cache but I had some problems, primarily that my AdSense ads were not always showing. By the way the WP Rocket plugin is awesome to use. This helped me disable lazy loading on the first image for pagespeed insights. thanks again for sharing
Hey Redoy, happy it helped!
James, you’re my hero! Worked like a charm. I’ve been trying to do this literally all day to improve my Google PageSpeed score and you nailed it. I wish WP Rocket would offer a checkbox in the admin panel or something out of the box. Thank you soo much its working great!!
Hey Kate! Awesome! I wrote this to scratch my own itch, our site was really well optimized except our contentful paint score was hurting due to our lazy loading plugin preventing the top-most image from loading. Glad I’m not the only one who found this useful 🙂
Hi, I’m looking for something like this.
Unfortunately it doesn’t work for me.
I tried in the posts, nothing. Changed the css class, nothing.
Could you write something to exclude the first image in the whole site?
Hi Marco!
Right click on an image in your post and click “Inspect”. Look at the CSS classes.
This only works if the CSS classes you’ve entered matches the image settings that your posts use.
If they all have different alignments and different sizes, you will need to modify this code to accomodate that.
For example, on this post on JamesParsons.com, the class says: “alignleft size-full”. So, I would change the code where it says “alignnone size-full” and replace alignnone with alignleft. Add it to functions.php and then it will work.
If you use a drag-and-drop website builder or any sort of custom code to insert your images, this may not work for you.
You may need to hire a developer to inspect this code for you and adapt it to your specific website.
This was actually developed for a different website of mine and for my clients, but I just implemented it on this site so you can see it in action. Notice how the first image in this post is not being lazyloaded, and how the PageSpeed score improved.
Hi James!
How can use your code for all page (category,post,…)
🙏
Hi Amir,
You’d want to change line 6 where it says: is_single()
Something like this would work for (most) all pages:
if ( is_single() || is_page() || is_category() || is_author() ) {
I’m missing some, like is_archive, is_attachment, etc. You may want to avoid using it on some page types, so this may be the best way to do it.
Of course, still make sure line 31 is looking for the correct class names of your first image on each page.
You may want to modify this or add multiple attributes to match multiple page types, in which case you’d copy and paste line 31 and edit the class names.
Hi, i haven’t WP Rocket plugin, and i try to modify your second function with this, but i doesn’t work.
function remove_lazy_loading_for_specific_class( $attributes ) {
$attributes[] = ‘class=”aligncenter size-full remove-lazy”‘;
return $attributes;
}
add_filter( ‘wp_img_tag_add_loading_attr’, ‘remove_lazy_loading_for_specific_class’ );
So i need to remove the lazy load only on image with class “class=”alignnone size-full remove-lazy”. How can I do that? Thanks 🙂
You have to change it on line 16 as well 🙂
thank you for responding
I shared your idea with some plugin developers and get good feedback from Autoptimize developer.
https://wordpress.org/support/topic/disable-lazy-load-image-for-the-first-image-above-the-fold/
also :
https://wordpress.org/support/topic/exclude-the-first-x-images-from-the-lazy-loading/
https://wordpress.org/support/topic/disable-lazy-load-image-for-the-first-image-above-the-fold-2/
Thanks for sharing! This is good to know for Autoptimize users.
I’ve implemented this on my site.
My only concern is
For my images, the image class was like: “alignnone wp-image-157580 size-full”
this is being replaced with “alignnone size-full remove-lazy”
Where my “wp-image-157580” attribute is getting lost. Can we have a fix for this?
Hi Vijay!
This should be easy to change. Simply change “size-full” in the code to “wp-image-157580”.
If that “wp-image-157580” value is different for every image, you might want to consider finding the CSS for those classes and copy it a new class in your stylesheet called “.size-full”.
This would be much easier than hacking up this code.
Hey James, finally found something I have been looking for, btw I don’t have WP rocket installed, but I do have Autooptimize.
The problem is Google’s PSI is telling me my LCP is not good because the WordPress featured image is lazy loaded, can you help me with the solution for this please?
Drop me an email, I’ll see what I can do to help.
Hello,
Quick question..is this only for WP Rocket users? I currently use SG Optimizer.
Thanks so much!
Yes, only for WP Rocket.
I’m sure theres a way to do it with SG Optimizer too. If you can, I recommend upgrading to WP Rocket, though!
Hi James
Thank you so much for your code, I snippet the code on my site, first it looked very well but after some time I realized that code causes some error on products page. Can you please update the code. Maybe it has some conflict with some plugins.
Thank you so much
Hey Ommy!
It’s hard to say what the error is or which of your plugins are conflicting.
Could you share more details?